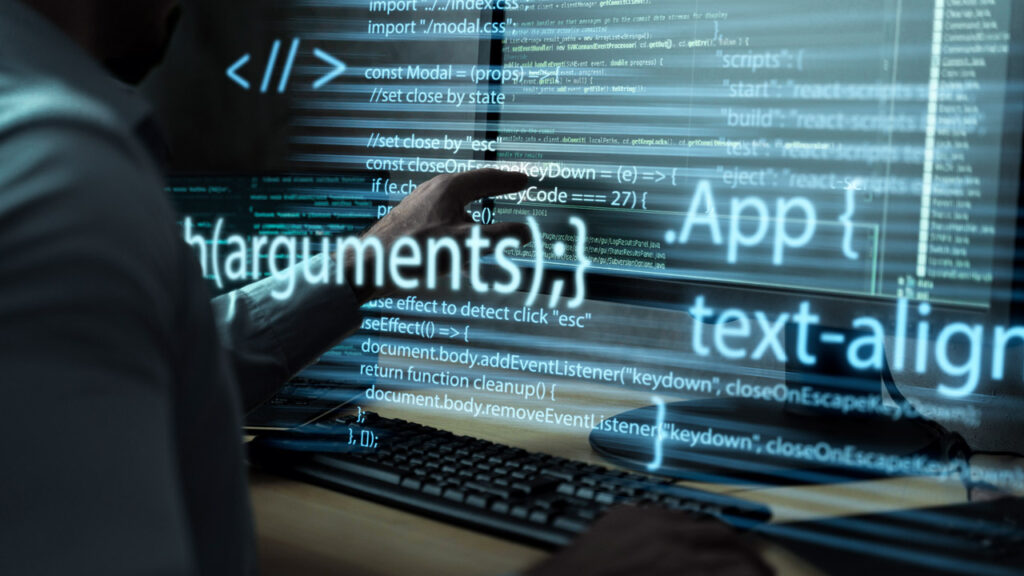
In the process of scoping CSS library like Bootstrap, the goal is to apply all the styles only within a specific scope (like a wrapper class) without affecting the rest of the page. CSS scope technique is useful when you want to avoid design conflicts between styles from different libraries or when embedding a library in a larger project with its styles.
Table of Contents
What is the scope CSS library?
Scope CSS library means limiting the styles defined in that library to apply only to a specific section of your web page, usually by adding a custom class or identifier. This ensures that the library’s styles (like Bootstrap or any other CSS framework) do not interfere with or overwrite the global styles of your website or other parts of your project.
Why Scope CSS Library?
- Avoid Conflicts: Scoping helps prevent style conflicts between different CSS frameworks or custom styles that might unintentionally override each other.
- Maintain Isolation: If you’re using multiple CSS frameworks or integrating third-party components into your project, scoping keeps their styles isolated.
- Reuse: In large applications, you might want different parts of your site to use different styling rules without interfering with each other.
There are several ways to scope CSS library. I will share the most reliable and robust approaches, starting from simple solutions to more advanced techniques.
Approach 1: Prefixing Classes with a Wrapper (Manual)
The simplest way to scope CSS library is by manually prefixing all CSS selectors with a wrapper class. For example, by adding .wpfrank
as a prefix, you scope CSS to only elements inside this class.
<style>
.wpfrank .container {
margin: 0 auto;
}
.wpfrank .btn {
padding: 10px;
}
</stle>
<div class="wpfrank">
<div class="container">
<button class="btn btn-primary">Click Me</button>
</div>
</div>
Approach 2: Using a PostCSS Plugin (Automated and More Robust)
A more scalable way is to use PostCSS with the postcss-prefixwrap plugin, which automatically scopes CSS rules. This is ideal for large libraries like Bootstrap and provides more flexibility.
Approach 3: Use Shadow DOM (Advanced)
The Shadow DOM is a web standard that encapsulates the styles of web components. This is the cleanest and most isolated way to scope CSS styles, but it requires the use of web components.
<div id="my-shadow-container"></div>
<script>
const container = document.querySelector('#my-shadow-container');
const shadowRoot = container.attachShadow({ mode: 'open' });
shadowRoot.innerHTML = `
<style>
@import 'bootstrap.css'; /* Importing bootstrap styles inside the shadow DOM */
</style>
<div class="container">
<button class="btn btn-primary">Click Me</button>
</div>
`;
</script>
Approach 4: Iframe (Isolated Environment)
Using an iframe is another way to completely isolate styles. The content inside the iframe won’t be affected by the parent document’s CSS, and vice versa.
<iframe src="iframe-content.html"></iframe>
<link rel="stylesheet" href="bootstrap.css">
<div class="container">
<button class="btn btn-primary">Click Me</button>
</div>
In this article, we will explain Approach 2 for scope CSS in detail with step by step process:
Scope CSS using the PostCSS Plugin
Here’s a complete step-by-step guide, including installing Node.js, setting up PostCSS, and running the script to scope your CSS file.
Step 1: Install Node.js
Node.js is a runtime environment that allows you to run JavaScript on your computer and manage packages through npm (Node Package Manager).
1.1 Download and Install Node.js
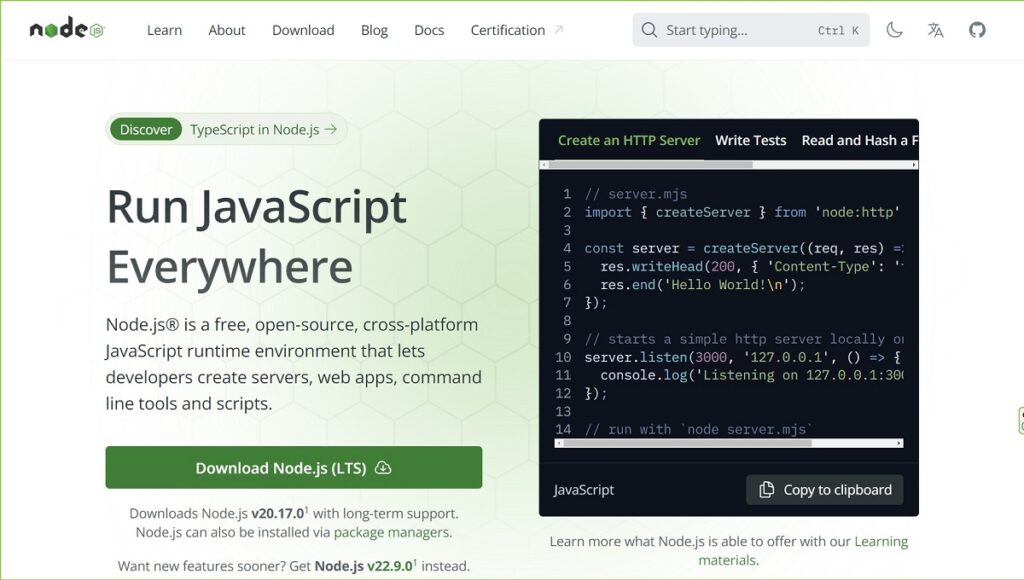
- Go to the official Node.js website: https://nodejs.org/.
- Download the LTS (Long Term Support) version suitable for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the on-screen instructions.
- During installation, make sure to check the option to “Install npm” (which is included with Node.js).
1.2 Verify the Installation
After installation, open your terminal or command prompt and type the following commands to verify that Node.js and npm were installed correctly:
node -v
This command should return the installed Node.js version (e.g., v20.17.0
).
npm -v
This command should return the installed npm version (e.g., v10.8.2
).
Step 2: Set Up a Project for Scoping Bootstrap
Now that Node.js is installed, we’ll set up a project folder, and install PostCSS, and the required plugins for scoping the Bootstrap CSS.
2.1 Create a Project Folder
Create a new folder where you’ll work on scoping the Bootstrap CSS:
mkdir bootstrap-scoped
cd bootstrap-scoped
2.2 Initialize npm
Inside your project folder, run the following command to initialize an npm project. This will create a package.json
file where your dependencies will be listed.
npm init -y
Step 3: Install PostCSS and Plugins
Next, you need to install PostCSS and the postcss-prefixwrap
plugin, which will automatically add the class prefix to all CSS selectors.
3.1 Install PostCSS and Autoprefixer
Run the following command to install PostCSS and the autoprefixer plugin (which is commonly used alongside PostCSS):
npm install postcss autoprefixer --save-dev
3.2 Install the postcss-prefixwrap Plugin
Run the following command to install the postcss-prefixwrap
plugin, which is responsible for scoping the CSS:
npm install postcss-prefixwrap --save-dev
Step 4: Configure PostCSS
Now that PostCSS and the necessary plugins are installed, you need to create a configuration file to tell PostCSS how to process your CSS.
Create postcss.config.js – In the root of your project folder, create a file called postcss.config.js and add the following content to it:
module.exports = {
plugins: [
require('postcss-prefixwrap')('.wpfrank'), // Add your custom prefix here
require('autoprefixer') // Autoprefixer can be used to add browser vendor prefixes (optional)
]
};
This configuration tells PostCSS to use the postcss-prefixwrap plugin with the class .wpfrank as a prefix, and the optional autoprefixer plugin to ensure compatibility with different browsers.
Step 5: Prepare the Bootstrap CSS
Download the bootstrap.css file if you haven’t already. You can get it from Bootstrap’s official website or use your existing bootstrap.css. To Scope CSS of the Bootstrap library, you need a non-minified file.
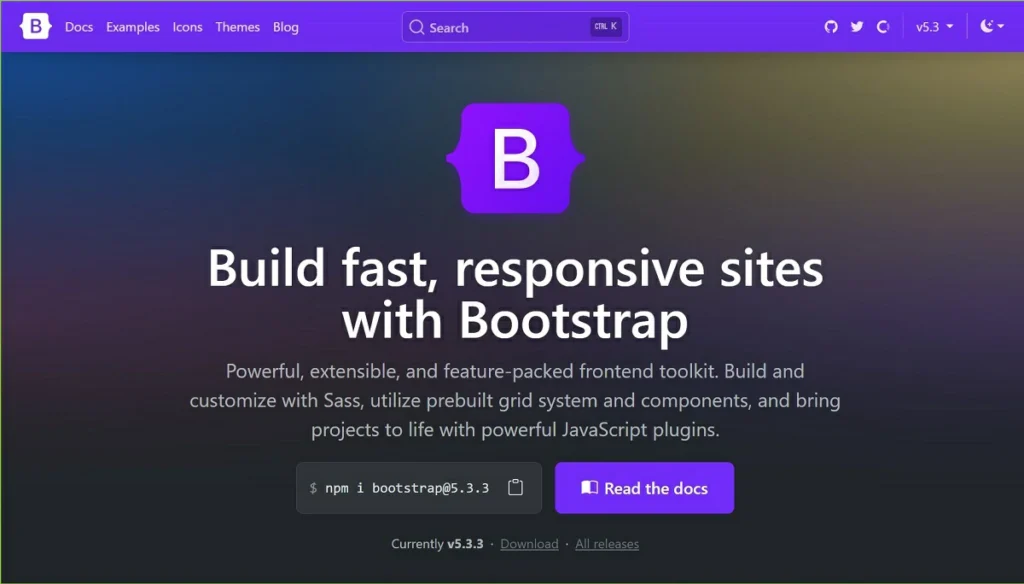
Place the bootstrap.css file in your project folder (e.g., bootstrap-scoped).
Step 6: Create the PostCSS Build Script
In your package.json file, add a custom script that runs PostCSS to process your bootstrap.css and generate the scoped version.
Open package.json
and find the "scripts"
section. Update it like this:
{
"scripts": {
"build-css": "postcss bootstrap.css -o bootstrap-scoped.css"
}
}
This script tells PostCSS to process bootstrap.css and output a new file called bootstrap-scoped.css with the prefix applied.
Step 7: Run PostCSS to Generate Scoped CSS
Now that everything is set up, you can run the build script to generate the scoped version of Bootstrap.
7.1 Run the Build Script
Run the following command to execute the PostCSS build script:
npm run build-css
This command will process your bootstrap.css file and create a new file called bootstrap-scoped.css in your project folder, with all CSS selectors prefixed by .wpfrank. So, you will get a scope CSS library as a result.
Step 8: Use the Scope CSS in Your Project
Once the scope CSS (bootstrap-scoped.css) is generated, you can include it in your project and wrap the relevant HTML in the .wpfrank class.
8.1 Example Usage
<link rel="stylesheet" href="bootstrap-scoped.css">
<div class="wpfrank">
<div class="container">
<button class="btn btn-primary">Click Me</button>
</div>
</div>
Now, all Bootstrap styles are scoped under the .wpfrank class, preventing conflicts with other global styles.
Step 9: Optional – Watch for Changes
If you’re making ongoing changes to your scope CSS, you can add a watch command to process the CSS file whenever it changes automatically.
9.1 Add Watch Script
Update your package.json to include a watch script:
{
"scripts": {
"build-css": "postcss bootstrap.css -o bootstrap-scoped.css",
"watch-css": "postcss --watch bootstrap.css -o bootstrap-scoped.css"
}
}
9.2 Run the Watch Command
Now, you can run the following command to recompile the CSS whenever you modify bootstrap.css automatically:
npm run watch-css
Error and Troubleshooting
Error: npm error could not determine executable to run
The error message “npm error could not determine executable to run” indicates that npm could not find the executable to run the command, possibly due to an issue with the installation of PostCSS or its plugins.
Step 1: Check the PostCSS Installation
Ensure that postcss-cli is installed, as it is required to run PostCSS commands. Run the following command to install it:
npm install postcss-cli --save-dev
Step 2: Verify Plugins Installation
Ensure that the required PostCSS plugins (autoprefixer and postcss-prefixwrap) are installed:
npm install autoprefixer postcss-prefixwrap --save-dev
Step 3: Delete node_modules and package-lock.json
Sometimes, errors can occur due to incorrect or corrupt node_modules. To delete node_modules and package-lock.json, run the following commands in your Command Prompt:
rmdir /s /q node_modules
del package-lock.json
Step 4: Modify the package.json Script
Ensure that your package.json has the correct script and uses npx to run the local version of PostCSS:
{
"scripts": {
"build-css": "npx postcss bootstrap.css -o bootstrap-scoped.css"
}
}
Step 5: Try Running the Build Again
After completing the above steps, try running the command again:
npm run build-css
Step 6: Check the Log File
If the error persists, check the log file (C:\Users\Pc-New\AppData\Local\npm-cache_logs\2024-10-02T07_11_26_650Z-debug-0.log) to get more detailed information about what went wrong. The error log might contain useful hints such as missing dependencies, misconfigurations, or permissions issues.
If the error log contains specific errors, feel free to share them, and I can help you resolve them.
Conclusion
The best approach scope CSS depends on your use case:
- Manual Prefixing (Approach 1) is simple and effective for small to medium libraries or limited scope.
- PostCSS with prefixing (Approach 2) is more scalable and automated for large libraries.
- Shadow DOM (Approach 3) is the most robust but may require significant restructuring of your project.
- Iframe (Approach 4) is effective for complete isolation but can introduce complexity.
I recommend using PostCSS with postcss-prefixwrap (Approach 2) for a large library like Bootstrap as it automates the scoping process and is highly customizable. Let us know if you’d like help implementing any of these scope CSS approaches, any comment is appreciated!