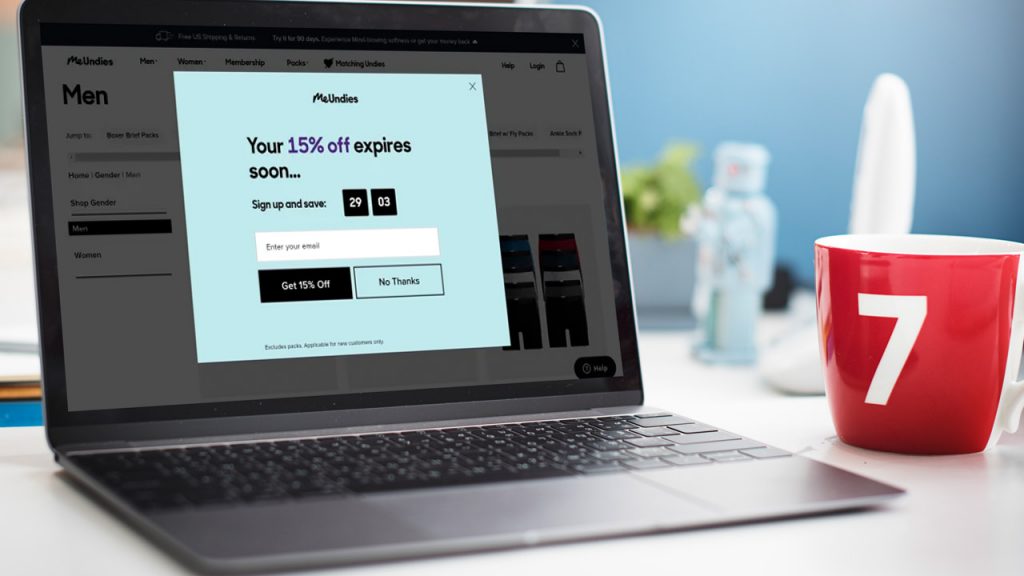
A web theme often makes it easier for users to notice modal popup windows because they match their design. HTML, CSS, and JavaScript are used to build modules. You can create lightboxes, user notifications, or custom content for your site by using Bootstrap’s JavaScript modal plugin. Modal popup windows usually lack navigation buttons and menu headings and have a different appearance from normal windows.
Table of Contents
Why you should use a modal popup on your website?
In one word, modals serve the purpose of focusing attention. Your website’s usability improves when you use modal windows. The modular dialog interrupts the user and demands action. Additionally, modular windows allow you to get rid of large elements that don’t need to be on the main page, saving you space.
Create Modal using Bootstrap 5
First, you need to download a stable version of the Bootstrap toolkit and always use the stable release of the third-party libraries for development. Currently, Bootstrap has a 5.1.3 major release and a stable version to work with.
After downloading the zip package unzip it on your computer, then you will get the bootstrap-5.1.3-dist folder. Create an index.html file, in that directory. Now, we will load two bootstrap-required libraries for modal in that file.
<link rel="stylesheet" href="css/bootstrap.css">
<script src="js/bootstrap.js" type="text/javascript"></script>
The above bootstrap.css file is required for design and the bootstrap.js file has all defined js functionality for Modal. Before loading the bootstrap.js file you need to load the latest jQuery library because every third-party library is dependent on the jQuery library.
<script src="js/jquery-3.6.0.js" type="text/javascript"></script>
So, our index.html file has the following HTML code:
<html lang="en-US">
<head>
<title>Bootstrap 5 Modal</title>
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" href="css/bootstrap.css">
<script src="js/jquery-3.6.0.js" type="text/javascript"></script>
<script src="js/bootstrap.js" type="text/javascript"></script>
<!-- Modal Java Script Functions -->
<script type="text/javascript">
$( document ).ready(function() {
//creating modal element object
var myModal = new bootstrap.Modal(document.getElementById('MyModal'), {
backdrop: true,
keyboard: true,
focus: true,
});
myModal.show(); // this will show modal on page load
});
</script>
</head>
<body>
<!-- Modal Trigger Button -->
<button type="button" class="btn btn-primary m-4" data-bs-toggle="modal" data-bs-target="#MyModal">
Launch Modal
</button>
<!-- Modal Body Start-->
<div class="modal fade" id="MyModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal Title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<p>Modal Body</p>
<p>...</p>
<p>...</p>
<p>...</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<!-- Modal Body End-->
</body>
</html>
In the HTML body code, we have two parts. The first one is a Modal Trigger Button, which will be used to launch the modal when you click on that button.
The second part is the Modal Body, this part contains the modal header, modal body, and modal footer. We used the modal body div id=”MyModal” to connect with the javascript function. The MyModal ID will be used to trigger the modal popup when you load the page or when you click on the button.
<!-- Modal Java Script Functions -->
<script type="text/javascript">
$( document ).ready(function() {
//creating modal element object
var myModal = new bootstrap.Modal(document.getElementById('MyModal'), {
backdrop: true,
keyboard: true,
focus: true,
});
myModal.show(); // this will show modal on page load
});
</script>
In the above javascript function, we created a myModal as a Bootstrap Modal object. This modal object will be used to perform all modal events like the show, hide, or close the modal. Like below js code will show the modal when you click on the Modal Trigger Button “Launch Modal“.
myModal.show();
If you want the same on your own then download the whole working package using the below link. You can use an HTML code validator to test code syntax and errors.
Hope you find this tutorial useful for your practical usage. If you have any queries related to modal pop customization comment down below, I will try my best to help you.
Cheers!!!